Template Class for reading a row. More...
#include "rdm_db_read_row.h"
Public Member Functions |
|
RDM_RETCODE | fetch (direction dir, bool first_or_last) |
Fetch a row. More... |
|
read_row & | operator= (const read_row &r) |
read_row (const read_row &r) | |
read_row () | |
~read_row () | |
uint32_t | init_tables_to_write_lock (RDM_TABLE_ID *tables) |
IDs of the tables where rows are inserted. More... |
|
uint32_t | init_tables_to_read_lock (RDM_TABLE_ID *tables) |
IDs of the tables where rows are read. More... |
|
RDM_RETCODE | init (RDM_DB db) |
Initialize this object. More... |
|
void | reset (void) |
Reset this object. More... |
|
template<class TARGET_ROW_T > | |
RDM_RETCODE | bind_row (TARGET_ROW_T *target_row) |
Bind a row. More... |
|
RDM_RETCODE | get_rows (void) |
Get rows. More... |
|
RDM_RETCODE | get_rows_by_key (RDM_KEY_ID key_id) |
Get rows by key. More... |
|
template<class KEY_T > | |
RDM_RETCODE | get_rows_by_key (RDM_KEY_ID key_id, const KEY_T *startValue, const KEY_T *endValue) |
Get rows by key within a key range. More... |
|
template<class KEY_T > | |
RDM_RETCODE | get_rows_by_key (RDM_KEY_ID key_id, const KEY_T *value) |
Get rows by key for a give key value. More... |
|
template<class KEY_T > | |
RDM_RETCODE | get_rows_from_key (RDM_KEY_ID key_id, const KEY_T *startValue) |
Get rows by key within a key range that is open at the end. More... |
|
template<class KEY_T > | |
RDM_RETCODE | get_rows_to_key (RDM_KEY_ID key_id, const KEY_T *endValue) |
Get rows by key within a key range that is open at the beginning. More... |
|
RDM_RETCODE | get_rows_by_key (RDM_KEY_ID key_id, const RDM_RANGE_KEY *startValue, const RDM_RANGE_KEY *endValue) |
Get rows by key within a key range. More... |
|
RDM_RETCODE | get_rows_by_key (RDM_KEY_ID key_id, const RDM_RANGE_KEY *value) |
Get rows by key for a give key value. More... |
|
RDM_RETCODE | get_rows_from_key (RDM_KEY_ID key_id, const RDM_RANGE_KEY *startValue) |
Get rows by key within a key range that is open at the end. More... |
|
RDM_RETCODE | get_rows_to_key (RDM_KEY_ID key_id, const RDM_RANGE_KEY *endValue) |
Get rows by key within a key range that is open at the beginning. More... |
|
RDM_RETCODE | get_rows_by_key (RDM_KEY_ID key_id, const RDM_SEARCH_KEY *startValue, const RDM_SEARCH_KEY *endValue) |
Get rows by key within a key range. More... |
|
RDM_RETCODE | get_rows_by_key (RDM_KEY_ID key_id, const RDM_SEARCH_KEY *value) |
Get rows by key for a give key value. More... |
|
RDM_RETCODE | get_rows_from_key (RDM_KEY_ID key_id, const RDM_SEARCH_KEY *startValue) |
Get rows by key within a key range that is open at the end. More... |
|
RDM_RETCODE | get_rows_to_key (RDM_KEY_ID key_id, const RDM_SEARCH_KEY *endValue) |
Get rows by key within a key range that is open at the beginning. More... |
|
RDM_RETCODE | get_rows_by_rtree_key (RDM_KEY_ID key_id, const RDM_RTREE_KEY *value) |
Get rows by an R-tree key. More... |
|
RDM_RETCODE | fetch_first (void) |
Fetch the first row. More... |
|
RDM_RETCODE | fetch_prev (void) |
Fetch the previouse row. More... |
|
RDM_RETCODE | fetch_next (void) |
Fetch the next row. More... |
|
RDM_RETCODE | fetch_last (void) |
Fetch the first row. More... |
|
Static Public Member Functions |
|
constexpr static int | number_of_tables_to_write_lock (void) |
Number of tables where rows are inserted. More... |
|
constexpr static int | number_of_tables_to_read_lock (void) |
Number of tables where rows are read. More... |
|
Data Fields |
|
RDM_DB | db |
RDM_CURSOR | cursor |
void * | target_row |
uint32_t | target_size |
Friends |
|
template<RDM_TABLE_ID any_table_id, class ANY_NEXT > | |
class | join_rows_to_many |
template<RDM_TABLE_ID any_table_id, class ANY_NEXT > | |
class | join_rows_to_one |
Detailed Description
template<RDM_TABLE_ID table_id>
class RDM::DB::read_row< table_id >
Template Class for reading a row.
Template class for reading rows from a table specifed by the template parameter table_id.
Initialize the class by calling init() with a valid database handle. Bind an application row buffer using bind_row(). A set of rows from the table specified by the template parameter table_id with a given order can be associated by calling any of the following methods: get_rows_by_key(), TBD.
The application row buffer will be populated when any of the navigational fetch methods are called such as fetch_first(), fetch_prev(), fetch_next(), or fetch_last().
This class can be used in a chain of template classes for doing a join or a series of nested joins where this class is the last class in the chain. When this class is used in a chain of classes the navigational fetch methods for this class should normally not be called directly. The application should instead call the navigational fetch methods of the first class the chain. The other classes in the chain will fetch from its next class when additional rows are needed. See RDM Database Query APIs for details.
- Template Parameters
-
table_id The ID of the table to read rows from
Constructor & Destructor Documentation
read_row() [1/2]
|
inline |
read_row() [2/2]
|
inline |
~read_row()
|
inline |
References RDM::DB::read_row< table_id >::cursor, and rdm_cursorFree().

Member Function Documentation
bind_row()
|
inline |
Bind a row.
Bind an application target row. For each row that is fetched one actual row in that set will be populated into the buffers specified here.
Only one buffer is provided here as opposed to other classes.
- Template Parameters
-
TARGET_ROW_T The type for the application target row struct definition
- Return values
-
sOKAY Normal, successful return.
- Parameters
-
[in] target_row The target the rows are fetched into
References sOKAY, RDM::DB::read_row< table_id >::target_row, and RDM::DB::read_row< table_id >::target_size.
fetch()
|
inline |
Fetch a row.
A generalized fetch which is the actual methode used by other classes when this class is set up in a chain.
- Return values
-
sOKAY Normal, successful return. sENDOFCURSOR Reached the end of the cursor collection. eINVITERATION Invalid attempt to move cursor before 'BeforeFirst' or past 'AfterLast'. eNOSTARTREAD A read operation was attempted when no rdm_dbStartSnapshot(), rdm_dbStartRead(), or rdm_dbStartUpdate() is active. eNOTLOCKED Attempt to access a table for reading or update without proper locks. eDBNOTOPEN Database not open. eCATMISMATCH Catalog in memory does not match Catalog on disk. eNOCURRENTROW The cursor is not positioned to a valid row. eOWNERDELETED The owner row for a set cursor has been deleted. eSINGLETONDELETED The row for a singleton cursor has been deleted.
- Parameters
-
[in] dir The direction we are fetching [in] first_or_last Wheter we are fetching one of the extremes such as the first or the last as opposed to the next or the previous
References RDM::DB::direction_forward, RDM::DB::read_row< table_id >::fetch_first(), RDM::DB::read_row< table_id >::fetch_last(), RDM::DB::read_row< table_id >::fetch_next(), and RDM::DB::read_row< table_id >::fetch_prev().
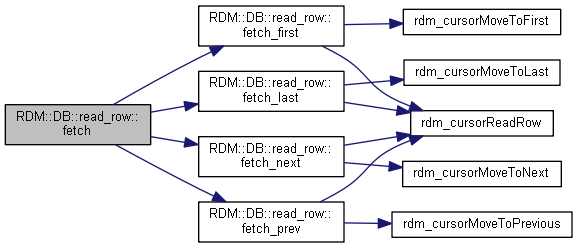
fetch_first()
|
inline |
Fetch the first row.
Fetch the first row from the actual table. This navigation is done independent of any previously set position. A new position will be establshed for a successfull return.
If a row has been bound using bind_row() the row will be read and poputated into the buffers provided.
- Return values
-
sOKAY Normal, successful return. sENDOFCURSOR Reached the end of the cursor collection. eINVITERATION Invalid attempt to move cursor before 'BeforeFirst' or past 'AfterLast'. eNOSTARTREAD A read operation was attempted when no rdm_dbStartSnapshot(), rdm_dbStartRead(), or rdm_dbStartUpdate() is active. eNOTLOCKED Attempt to access a table for reading or update without proper locks. eDBNOTOPEN Database not open. eCATMISMATCH Catalog in memory does not match Catalog on disk. eNOCURRENTROW The cursor is not positioned to a valid row. eOWNERDELETED The owner row for a set cursor has been deleted. eSINGLETONDELETED The row for a singleton cursor has been deleted.
References RDM::DB::read_row< table_id >::cursor, rdm_cursorMoveToFirst(), rdm_cursorReadRow(), sOKAY, and RDM::DB::read_row< table_id >::target_size.
Referenced by RDM::DB::read_row< table_id >::fetch().
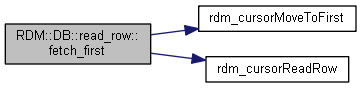

fetch_last()
|
inline |
Fetch the first row.
Fetch the first row from the actual table. This navigation is done independent of any previously set position. A new position will be establshed for a successfull return.
If a row has been bound using bind_row() the row will be read and poputated into the buffers provided.
- Return values
-
sOKAY Normal, successful return. sENDOFCURSOR Reached the end of the cursor collection. eINVITERATION Invalid attempt to move cursor before 'BeforeFirst' or past 'AfterLast'. eNOSTARTREAD A read operation was attempted when no rdm_dbStartSnapshot(), rdm_dbStartRead(), or rdm_dbStartUpdate() is active. eNOTLOCKED Attempt to access a table for reading or update without proper locks. eDBNOTOPEN Database not open. eCATMISMATCH Catalog in memory does not match Catalog on disk. eNOCURRENTROW The cursor is not positioned to a valid row. eOWNERDELETED The owner row for a set cursor has been deleted. eSINGLETONDELETED The row for a singleton cursor has been deleted.
References RDM::DB::read_row< table_id >::cursor, rdm_cursorMoveToLast(), rdm_cursorReadRow(), sOKAY, and RDM::DB::read_row< table_id >::target_size.
Referenced by RDM::DB::read_row< table_id >::fetch().
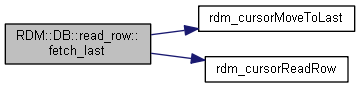

fetch_next()
|
inline |
Fetch the next row.
Fetch the next row from the actual table. This navigation is done based on a previously set position and a new position will be establshed for a successfull return.
If a row has been bound using bind_row() the row will be read and poputated into the buffers provided.
- Return values
-
sOKAY Normal, successful return. sENDOFCURSOR Reached the end of the cursor collection. eINVITERATION Invalid attempt to move cursor before 'BeforeFirst' or past 'AfterLast'. eNOSTARTREAD A read operation was attempted when no rdm_dbStartSnapshot(), rdm_dbStartRead(), or rdm_dbStartUpdate() is active. eNOTLOCKED Attempt to access a table for reading or update without proper locks. eDBNOTOPEN Database not open. eCATMISMATCH Catalog in memory does not match Catalog on disk. eNOCURRENTROW The cursor is not positioned to a valid row. eOWNERDELETED The owner row for a set cursor has been deleted. eSINGLETONDELETED The row for a singleton cursor has been deleted.
References RDM::DB::read_row< table_id >::cursor, rdm_cursorMoveToNext(), rdm_cursorReadRow(), sOKAY, and RDM::DB::read_row< table_id >::target_size.
Referenced by RDM::DB::read_row< table_id >::fetch().
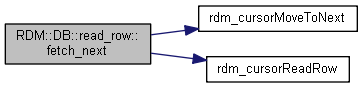

fetch_prev()
|
inline |
Fetch the previouse row.
Fetch the previous row from the actual table. This navigation is done based on a previously set position and a new position will be establshed for a successfull return.
If a row has been bound using bind_row() the row will be read and poputated into the buffers provided.
- Return values
-
sOKAY Normal, successful return. sENDOFCURSOR Reached the end of the cursor collection. eINVITERATION Invalid attempt to move cursor before 'BeforeFirst' or past 'AfterLast'. eNOSTARTREAD A read operation was attempted when no rdm_dbStartSnapshot(), rdm_dbStartRead(), or rdm_dbStartUpdate() is active. eNOTLOCKED Attempt to access a table for reading or update without proper locks. eDBNOTOPEN Database not open. eCATMISMATCH Catalog in memory does not match Catalog on disk. eNOCURRENTROW The cursor is not positioned to a valid row. eOWNERDELETED The owner row for a set cursor has been deleted. eSINGLETONDELETED The row for a singleton cursor has been deleted.
References RDM::DB::read_row< table_id >::cursor, rdm_cursorMoveToPrevious(), rdm_cursorReadRow(), sOKAY, and RDM::DB::read_row< table_id >::target_size.
Referenced by RDM::DB::read_row< table_id >::fetch().
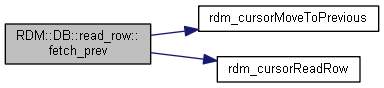

get_rows()
|
inline |
Get rows.
Set up this class to get the rows from a give table.
- Return values
-
sOKAY Normal, successful return. eINVTABID Invalid table id. eDBNOTOPEN Database not open. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRows().

get_rows_by_key() [1/7]
|
inline |
Get rows by key.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eISRTREE The key specified is an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Parameters
-
[in] key_id The key ID for which key to order the rows
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKey().

get_rows_by_key() [2/7]
|
inline |
Get rows by key within a key range.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eISRTREE The key specified is an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Template Parameters
-
KEY_T The key type for the key values provided
- Parameters
-
[in] key_id The key ID for which key to order the rows [in] startValue The value for the start of the range [in] endValue The value for the end of the range
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKeyInKeyRange().

get_rows_by_key() [3/7]
|
inline |
Get rows by key for a give key value.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eISRTREE The key specified is an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Template Parameters
-
KEY_T The key type for the key value provided
- Parameters
-
[in] key_id The key ID for which key to order the rows [in] value The value to select
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKeyInKeyRange().

get_rows_by_key() [4/7]
|
inline |
Get rows by key within a key range.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eISRTREE The key specified is an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Parameters
-
[in] key_id The key ID for which key to order the rows [in] startValue The value for the start of the range [in] endValue The value for the end of the range
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKeyInRangeKeyRange().

get_rows_by_key() [5/7]
|
inline |
Get rows by key for a give key value.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eISRTREE The key specified is an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Parameters
-
[in] key_id The key ID for which key to order the rows [in] value The value to select
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKeyInRangeKeyRange().

get_rows_by_key() [6/7]
|
inline |
Get rows by key within a key range.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eISRTREE The key specified is an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Parameters
-
[in] key_id The key ID for which key to order the rows [in] startValue The value for the start of the range [in] endValue The value for the end of the range
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKeyInSearchKeyRange().

get_rows_by_key() [7/7]
|
inline |
Get rows by key for a give key value.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eISRTREE The key specified is an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Parameters
-
[in] key_id The key ID for which key to order the rows [in] value The value to select
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKeyInSearchKeyRange().

get_rows_by_rtree_key()
|
inline |
Get rows by an R-tree key.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eNOTRTREE The key specified is not an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Parameters
-
[in] key_id The key ID for which key to order the rows [in] value The value for the end of the range
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKeyInRtreeKeyRange().

get_rows_from_key() [1/3]
|
inline |
Get rows by key within a key range that is open at the end.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eISRTREE The key specified is an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Template Parameters
-
KEY_T The key type for the key value provided
- Parameters
-
[in] key_id The key ID for which key to order the rows [in] startValue The value for the start of the range
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKeyInKeyRange().

get_rows_from_key() [2/3]
|
inline |
Get rows by key within a key range that is open at the end.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eISRTREE The key specified is an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Parameters
-
[in] key_id The key ID for which key to order the rows [in] startValue The value for the start of the range
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKeyInRangeKeyRange().

get_rows_from_key() [3/3]
|
inline |
Get rows by key within a key range that is open at the end.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eISRTREE The key specified is an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Parameters
-
[in] key_id The key ID for which key to order the rows [in] startValue The value for the start of the range
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKeyInSearchKeyRange().

get_rows_to_key() [1/3]
|
inline |
Get rows by key within a key range that is open at the beginning.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eISRTREE The key specified is an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Template Parameters
-
KEY_T The key type for the key value provided
- Parameters
-
[in] key_id The key ID for which key to order the rows [in] endValue The value for the end of the range
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKeyInKeyRange().

get_rows_to_key() [2/3]
|
inline |
Get rows by key within a key range that is open at the beginning.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eISRTREE The key specified is an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Parameters
-
[in] key_id The key ID for which key to order the rows [in] endValue The value for the end of the range
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKeyInRangeKeyRange().

get_rows_to_key() [3/3]
|
inline |
Get rows by key within a key range that is open at the beginning.
Set up this class to get the rows in key order.
- Return values
-
sOKAY Normal, successful return. eDBNOTOPEN Database not open. eINVKEYID Invalid key id. eISRTREE The key specified is an rtree index. ePRECOMMITTED A precommitted transaction must be committed or rolled back before further operations on this database are allowed.
- Parameters
-
[in] key_id The key ID for which key to order the rows [in] endValue The value for the end of the range
References RDM::DB::read_row< table_id >::cursor, RDM::DB::read_row< table_id >::db, and rdm_dbGetRowsByKeyInSearchKeyRange().

init()
|
inline |
Initialize this object.
Call this method before sending it any data values, ranges, or statistics.
- Return values
-
sOKAY Normal, successful return.
- Parameters
-
db [IN] Use this database for chained classes that need to insert rows
References RDM::DB::read_row< table_id >::db, and sOKAY.
init_tables_to_read_lock()
|
inline |
init_tables_to_write_lock()
|
inline |
number_of_tables_to_read_lock()
|
inlinestaticconstexpr |
number_of_tables_to_write_lock()
|
inlinestaticconstexpr |
operator=()
|
inline |
reset()
|
inline |
Reset this object.
Calling this method to discard all internally kept data values, ranges, and statistics that have not yet been persisted and start over from scratch.
Any alternative implementation of this method in a sub class would call this method to reset the next class.
References RDM::DB::read_row< table_id >::cursor, and rdm_cursorMoveToBeforeFirst().

Friends And Related Function Documentation
join_rows_to_many
|
friend |
join_rows_to_one
|
friend |
Field Documentation
cursor
RDM_CURSOR RDM::DB::read_row< table_id >::cursor |
The cursor we use for navigating and reading rows
Referenced by RDM::DB::read_row< table_id >::fetch_first(), RDM::DB::read_row< table_id >::fetch_last(), RDM::DB::read_row< table_id >::fetch_next(), RDM::DB::read_row< table_id >::fetch_prev(), RDM::DB::read_row< table_id >::get_rows(), RDM::DB::read_row< table_id >::get_rows_by_key(), RDM::DB::read_row< table_id >::get_rows_by_rtree_key(), RDM::DB::read_row< table_id >::get_rows_from_key(), RDM::DB::read_row< table_id >::get_rows_to_key(), RDM::DB::read_row< table_id >::reset(), and RDM::DB::read_row< table_id >::~read_row().
db
RDM_DB RDM::DB::read_row< table_id >::db |
The database we use for reading
Referenced by RDM::DB::read_row< table_id >::get_rows(), RDM::DB::read_row< table_id >::get_rows_by_key(), RDM::DB::read_row< table_id >::get_rows_by_rtree_key(), RDM::DB::read_row< table_id >::get_rows_from_key(), RDM::DB::read_row< table_id >::get_rows_to_key(), and RDM::DB::read_row< table_id >::init().
target_row
void* RDM::DB::read_row< table_id >::target_row |
The target row we write eche row we read into
Referenced by RDM::DB::read_row< table_id >::bind_row().
target_size
uint32_t RDM::DB::read_row< table_id >::target_size |
The size of the target row
Referenced by RDM::DB::read_row< table_id >::bind_row(), RDM::DB::read_row< table_id >::fetch_first(), RDM::DB::read_row< table_id >::fetch_last(), RDM::DB::read_row< table_id >::fetch_next(), and RDM::DB::read_row< table_id >::fetch_prev().
The documentation for this class was generated from the following file: