hash.h File Reference
#include "psptypes.h"
Include dependency graph for hash.h:
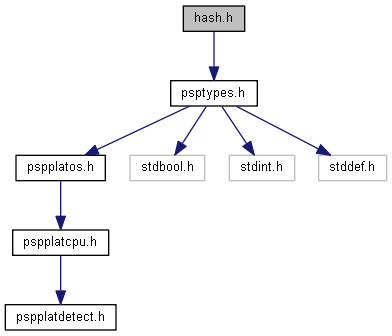
Data Structures |
|
struct | HASHCTX |
Macros |
|
#define | HASH_VALUE uint64_t |
#define | RDM_NUM_HASH_NUMBERS 8 |
Functions |
|
void | hashFcnStart (HASHCTX *hashfcn) |
void | hashFcnEmit (HASHCTX *hashfcn, uint8_t ch) |
uint64_t | hashFcnFinish (const HASHCTX *hashfcn) |
uint64_t | hashValue (uint8_t *values, uint32_t size) |
Macro Definition Documentation
HASH_VALUE
#define HASH_VALUE uint64_t |
RDM_NUM_HASH_NUMBERS
#define RDM_NUM_HASH_NUMBERS 8 |
Function Documentation
hashFcnEmit()
|
inline |
hashFcnFinish()
|
inline |
hashFcnStart()
|
inline |
References HASHCTX::hash, and HASHCTX::offset.
Referenced by hashValue().
Here is the caller graph for this function:

hashValue()
|
inline |
- Examples
- cpp50Example_main.cpp.
References hashFcnEmit(), hashFcnFinish(), and hashFcnStart().
Here is the call graph for this function:
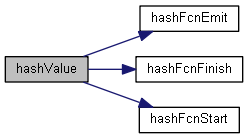
Definition: hash.h:24